Abstract:
C is a general-purpose computer programming language developed in 1972 by Dennis at the Bell Telephone Laboratories for use with the UNIX operating system.
Although C was designed for implementing system software, it is also widely used for developing portable application software. It is one of the most popular programming languages and there are very few computer architectures for which a C compiler does not exist. C has greatly influenced many other popular programming languages, most notably C++, which originally began as an extension to C.
C is sometimes referred to as a ``high-level assembly language.'' Some people think that's an insult, but it's actually a deliberate and significant aspect of the language. If you have programmed in assembly language, you'll probably find C very natural and comfortable. Although if you continue to focus too heavily on machine-level details, you'll probably end up with unnecessarily nonportable programs. If you haven't programmed in assembly language, you may be frustrated by C's lack of certain higher-level features. In either case, you should understand why C was designed this way: so that seemingly-simple constructions expressed in C would not expand to arbitrarily expensive (in time or space) machine language constructions when compiled. If you write a C program simply and succinctly, it is likely to result in a succinct, efficient machine language executable. If you find that the executable resulting from a C program is not efficient, it's probably because of something silly you did, not because of something the compiler did behind your back which you have no control over. In any case, there's no point in complaining about C's low-level flavor: C is what it is.
Introduction:
C is a very basic language. There are no frills, no GUIs, no Matrix processing abilities, very little file I/O support, etc.
Parts of a C program:
-
#include statements (preprocessor directives)
-
reserved words : (e.g., int, double, return, main, include, etc.)
-
variables : (similar to Matlab)
-
builtin functions (library functions) (printf, ...)
-
{} ( similar to Matlab start and end of functions)
-
main function
-
comments : (// single line, /* .... */ multiple line
Sample C Program:
The following is a "Design Pattern" for any basic C program:
#include
// tell C about printf
int // return value of main function
main() // where the program begins
{
printf("hello world\n");
return 0; // exit status of main program (could be any number
// that we agree means the program is finished)
}
Prospects:
why do we use C?
-
It was (and still is in some circumstances) the language of choice in Operating System Development (including all of Unix and Linux).
-
It allows you direct control over the very low level aspects of the computer.
-
Many legacy programs are written in C.
-
Most of the things you learn with C will be directly transferable to future programming languages.
-
Programs that are created with C run very quickly.
-
C has a syntax (and some semantics) very close to other programming languages like C++, Matlab, making the transition easy (easier...).
-
The programs you create in C will run "standalone". All of the programs we wrote in Matlab, need Matlab in order to work, and if you don't have access to Matlab, you are out of luck. C programs, once compiled into "executables", can be transferred to other (similar) machines, and run without the need for the source code.
-
Many of the codes you will use in your future work/studies will have been written in C. You should at the least, be able to read them. And hopefully, you will be able to maintain, modify, and update them.
Training Objective:
This short course is designed to ensure that students of Engineering College with academic Capabilities will have the skill set needed to deal with the challenges involved in real-world Programming using c, Advanced C or System C, to meet the needs of industries both today and in the future.
The course is taught mainly using the gcc compiler, with Linux operating system
Prerequisite:
A prior knowledge of a basic Linux commands, general understanding about operating system concepts is assumed.
Agenda:
-
There would be 17 sessions of 2 hours each.
-
There would be 20 lab sessions of 6 hours each.
-
After this Module is over, you should be:
-
Comfortable with respect to programming in C.
-
Able to Implement and use Data Structures using C.
-
Proficient in Advanced C.
-
Having good understanding about gcc compiler.
-
Able to debug complex C programs using gdb.
-
Ready for System Programming using C
-
Adept with Project Management tools.
-
Efficient with Source code management tools.
Training Topics in Brief:
-
Introduction and Programming Strategies
-
Features of C language, Broad structure of C.
-
Understanding C syntax, Data types, Escape sequences.
-
Operators, C conventions, Error Handling.
-
Some Programming Language Notes.
-
Structured Programming Strategies
-
Decision Making and Looping
-
Decision Structures
-
Looping Structures.
-
Break and Continue statements.
-
Functions
-
Function prototypes, function calling, function body, Arguments, by value and by Reference, Steps Involved In invoking a function, Recursive Functions, Types of functions, Functions Returning Pointers.
-
Arrays, Structures and Unions
-
Definition of Arrays, Comparison between arrays and pointers, Single Dimension Array, Two Dimensional Arrays, Structures & Unions, Memory layout, Bit fields in structure, functions and structures
-
Pointers
-
Declaration of pointers, Rules valid for pointers, Pointer usage, Pointers, addressing, referencing and dereferencing, Passing pointers to a function, Strings with Pointers.
-
String IO
-
Accessing individual characters, Printing strings with printf, Printing strings with puts, Inputting strings with scanf, Inputting strings with gets, String Library (strcpy(), strcat(), strcmp(), strchr(), strstr(), spintf(), sscanf(), atoi()).
-
Memory Allocation
-
Overview of memory management, Allocating new heap memory, _ Deallocating heap memory, Checking for successful memory allocation, Memory errors, Using memory you don’t own, Faulty heap management.
-
Operation in C
-
Files, File naming, Opening a file, Writing and reading a file, Character input and output, Direct file input and output operations, Closing and flushing of files, Sequential and random files.
-
Data Structures
-
Array and pointer:
-
Array creation, Array notation (pointer, direct etc), Review of pointer fundamentals, Review of Pointer operations, Parameter passing as pointers.
-
Sorting and searching methods
-
Various sorting techniques, Bubble sort, Merge sort, Quick sort, various search techniques: Sequential search, Binary search, Radix search.
-
Stack and queue
-
Stack Fundamentals, Stack implementation, Queue Fundamentals, Queue Implementation.
-
Linked list Fundamentals
-
Link list basics, Elementary link list functions.
-
Linked list Advanced
-
Reversing link list (different methods), by swapping, By recursion, Doubly link list, Basics of doubly link list, Circular link list.
-
Trees and Dynamic Algorithms:
-
Introduction, Binary tree, Complementary binary tree, Extended 2-Tree representation, Minimum weighted Path Length algorithm, Huffman’s Encoding, Balanced Binary Tree, and Paged Binary tree, M-Way Search Tree, Red-Black Tree, Threaded Binary Tree.
Training and Project Resources click here...
EmbLogic is an ISO 9001:2008(QMS) (Quality Management System) Certified Company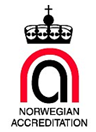